# Progress Indicator
This example show a graphical composant implemented in OpenGL that would not be easy to implement with raw RN components.
> N.B. the pie is transparent and the background is black with 50% opacity. That would easily allow to put this view on top of an image to show an image upload progress.
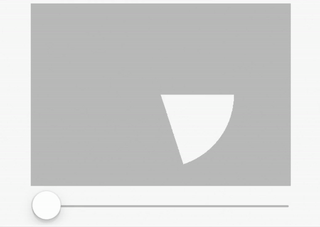
```html
```
*explicit props:*
```html
```
## Implementation
```js
const React = require("react-native");
const GL = require("gl-react-native");
const shaders = GL.Shaders.create({
pieProgress: {
frag: `
precision mediump float;
varying vec2 uv;
uniform vec4 colorInside, colorOutside;
uniform float radius;
uniform float progress;
uniform vec2 dim;
const vec2 center = vec2(0.5);
const float PI = acos(-1.0);
void main () {
vec2 norm = dim / min(dim.x, dim.y);
vec2 p = uv * norm - (norm-1.0)/2.0;
vec2 delta = p - center;
float inside =
step(length(delta), radius) *
step((PI + atan(delta.y, -delta.x)) / (2.0 * PI), progress);
gl_FragColor = mix(
colorOutside,
colorInside,
inside
);
}
`
}
});
class PieProgress extends React.Component {
render () {
const {
width,
height,
progress,
colorInside,
colorOutside,
radius
} = this.props;
return ;
}
}
PieProgress.defaultProps = {
colorInside: [0, 0, 0, 0],
colorOutside: [0, 0, 0, 0.5],
radius: 0.4
};
```
Note the usage of complex uniform types.
The JavaScript uniform:
```js
dim: [ width, height ]
```
is mapped to GLSL uniform:
```glsl
uniform vec2 dim;
```
where dim.x == width and dim.y == height.
Same mapping happens for the colors: `[1, 0.5, 0.2, 1]` mapped to a `vec4` will spread into {r,g,b,a}.
> Complex types in GLSL have "dynamic" properties:
vecN `v` can simultaneously be used using:
>```
v[0] v[1] v[2] v[3] // array notation
v.r v.g v.b v.a // color notation
v.x v.y v.z v.w // position notation
v.s v.t v.u v.v // coord notation
```